Организация интерфейса
Создадим теперь интерфейс, позволяющий конечному пользователю работать с объектами наших классов. Как всегда, интерфейс создавался вручную в режиме проектирования. На форме я создал меню с большим числом команд и инструментальную панель с 18 кнопками, команды которых повторяли основную команду меню. Описывать процесс создания интерфейса не буду - он подробно рассмотрен в предыдущей главе. Поскольку вся работа по созданию интерфейса транслируется в программный код формы, то просто приведу этот достаточно длинный текст почти без всяких купюр:
using System; using System.Drawing; using System.Collections; using System.ComponentModel; using System.Windows.Forms; using System.Data; using Shapes;
namespace Final { /// <summary> /// Эта форма обеспечивает интерфейс для создания, /// рисования, показа, перемещения, сохранения в списке /// и выполнения других операций над объектами семейства /// геометрических фигур. Форма имеет меню и /// инструментальные панели. /// </summary> public class Form1 : System.Windows.Forms.Form { //fields Graphics graphic; Brush brush, clearBrush; Pen pen, clearPen; Color color; Figure current; TwoWayList listFigure; private System.Windows.Forms.MainMenu mainMenu1; private System.Windows.Forms.ImageList imageList1; private System.Windows.Forms.ToolBar toolBar1; private System.Windows.Forms.MenuItem menuItem1; // аналогичные определения для других элементов меню private System.Windows.Forms.MenuItem menuItem35; private System.Windows.Forms.ToolBarButton toolBarButton1; // аналогичные определения для других командных кнопок private System.Windows.Forms.ToolBarButton toolBarButton18; private System.ComponentModel.IContainer components; public Form1() { InitializeComponent(); InitFields(); } void InitFields() { graphic = CreateGraphics(); color = SystemColors.ControlText; brush = new SolidBrush(color); clearBrush = new SolidBrush(SystemColors.Control); pen = new Pen(color); clearPen = new Pen(SystemColors.Control); listFigure = new TwoWayList(); current = new Person(20, 50, 50); } /// <summary> /// Clean up any resources being used. /// </summary> protected override void Dispose( bool disposing ) { if( disposing ) { if (components != null) { components.Dispose(); } } base.Dispose( disposing ); } #region Windows Form Designer generated code /// <summary> /// Required method for Designer support - do not modify /// the contents of this method with the code editor. /// </summary> private void InitializeComponent() { // Код, инициализирующий компоненты и построенный // дизайнером, опущен } #endregion /// <summary> /// Точка входа в приложение - процедура Main, /// запускающая форму /// </summary> [STAThread] static void Main() { Application.Run(new Form1()); } private void menuItem7_Click(object sender, System.EventArgs e) { createEllipse(); } void createEllipse() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create ellipse current = new Ellipse(50, 30, 180,180); } private void menuItem8_Click(object sender, System.EventArgs e) { createCircle(); } void createCircle() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create circle current = new Circle(30, 180,180); } private void menuItem9_Click(object sender, System.EventArgs e) { createLittleCircle(); } void createLittleCircle() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create littlecircle current = new LittleCircle(180,180); } private void menuItem10_Click(object sender, System.EventArgs e) { createRectangle(); } void createRectangle() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create rectangle current = new Rect(50, 30, 180,180); } private void menuItem11_Click(object sender, System.EventArgs e) { createSquare(); } void createSquare() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create square current = new Square(30, 180,180); } private void menuItem12_Click(object sender, System.EventArgs e) { createPerson(); } void createPerson() { //clear old figure if (current != null) current.Show(graphic, clearPen, clearBrush); //create person current = new Person(20, 180,180); } private void menuItem13_Click(object sender, System.EventArgs e) { showCurrent(); } void showCurrent() { //Show current current.Show(graphic, pen, brush); } private void menuItem14_Click(object sender, System.EventArgs e) { clearCurrent(); } void clearCurrent() { //Clear current current.Show(graphic, clearPen, clearBrush); } private void menuItem17_Click(object sender, System.EventArgs e) { incScale(); } void incScale() { //Increase scale current.Show(graphic, clearPen, clearBrush); current.Scale(1.5); current.Show(graphic, pen, brush); } private void menuItem18_Click(object sender, System.EventArgs e) { decScale(); } void decScale() { //Decrease scale current.Show(graphic, clearPen, clearBrush); current.Scale(2.0/3); current.Show(graphic, pen, brush); } private void menuItem19_Click(object sender, System.EventArgs e) { moveLeft(); } void moveLeft() { //Move left current.Show(graphic, clearPen, clearBrush); current.Move(-20,0); current.Show(graphic, pen, brush); } private void menuItem20_Click(object sender, System.EventArgs e) { moveRight(); } void moveRight() { //Move right current.Show(graphic, clearPen, clearBrush); current.Move(20,0); current.Show(graphic, pen, brush); } private void menuItem21_Click(object sender, System.EventArgs e) { moveTop(); } void moveTop() { //Move top current.Show(graphic, clearPen, clearBrush); current.Move(0,-20); current.Show(graphic, pen, brush); } private void menuItem22_Click(object sender, System.EventArgs e) { moveDown(); } void moveDown() { //Move down current.Show(graphic, clearPen, clearBrush); current.Move(0, 20); current.Show(graphic, pen, brush); } private void menuItem23_Click(object sender, System.EventArgs e) { //choose color ColorDialog dialog = new ColorDialog(); if (dialog.ShowDialog() ==DialogResult.OK) color =dialog.Color; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem24_Click(object sender, System.EventArgs e) { //Red color color =Color.Red; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem25_Click(object sender, System.EventArgs e) { //Green color color =Color.Green; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem26_Click(object sender, System.EventArgs e) { //Blue color color =Color.Blue; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem27_Click(object sender, System.EventArgs e) { //Black color color =Color.Black; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem28_Click(object sender, System.EventArgs e) { //Gold color color =Color.Gold; pen = new Pen(color); brush = new SolidBrush(color); } private void menuItem29_Click(object sender, System.EventArgs e) { //put_left: добавление фигуры в список listFigure.put_left(current); } private void menuItem30_Click(object sender, System.EventArgs e) { //put_right: добавление фигуры в список listFigure.put_right(current); } private void menuItem31_Click(object sender, System.EventArgs e) { //remove: удаление фигуры из списка if(!listFigure.empty()) listFigure.remove(); } private void menuItem32_Click(object sender, System.EventArgs e) { goPrev(); } void goPrev() { //go_prev: передвинуть курсор влево if(!(listFigure.Index == 1)) { listFigure.go_prev(); current = listFigure.item(); } } private void menuItem33_Click(object sender, System.EventArgs e) { goNext(); } void goNext() { //go_next: передвинуть курсор вправо if( !(listFigure.Index == listFigure.Count)) { listFigure.go_next(); current = listFigure.item(); } } private void menuItem34_Click(object sender, System.EventArgs e) { //go_first listFigure.start(); if(!listFigure.empty()) current = listFigure.item(); } private void menuItem35_Click(object sender, System.EventArgs e) { //go_last listFigure.finish(); if(!listFigure.empty()) current = listFigure.item(); } private void menuItem15_Click(object sender, System.EventArgs e) { showList(); } void showList() { //Show List listFigure.start(); while(listFigure.Index <= listFigure.Count) { current = listFigure.item(); current.Show(graphic,pen,brush); listFigure.go_next(); } listFigure.finish(); } private void menuItem16_Click(object sender, System.EventArgs e) { clearList(); } void clearList() { //Clear List listFigure.start(); while(!listFigure.empty()) { current = listFigure.item(); current.Show(graphic,clearPen,clearBrush); listFigure.remove(); } } private void Form1_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e) { if((current != null) && current.dragged_figure) { current.Show(graphic,clearPen,clearBrush); Point pt = new Point(e.X, e.Y); current.center_figure = pt; current.Show(graphic,pen,brush); } } private void Form1_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e) { current.dragged_figure = false; } private void Form1_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e) { Point mousePoint = new Point (e.X, e.Y); Rectangle figureRect = current.Region_Capture(); if ((current != null) && (figureRect.Contains(mousePoint))) current.dragged_figure = true; } protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { //show current figure current.Show(graphic, pen, brush); } private void toolBar1_ButtonClick(object sender, System.Windows.Forms.ToolBarButtonClickEventArgs e) { int buttonNumber = toolBar1.Buttons.IndexOf(e.Button); switch (buttonNumber) { case 0: createEllipse(); break; case 1: createCircle(); break; case 2: createLittleCircle(); break; case 3: createRectangle(); break; case 4: createSquare(); break; case 5: createPerson(); break; case 6: showCurrent(); break; case 7: clearCurrent(); break; case 8: showList(); break; case 9: clearList(); break; case 10: incScale(); break; case 11: decScale(); break; case 12: moveLeft(); break; case 13: moveRight(); break; case 14: moveTop(); break; case 15: moveDown(); break; case 16: goNext(); break; case 17: goPrev(); break; } } } }
Команд меню и кнопок в нашем интерфейсе много, поэтому много и обработчиков событий, что приводит к разбуханию кода. Но каждый из обработчиков событий довольно прост. Ограничусь кратким описанием главного меню:
- команды пункта главного меню Create позволяют создавать геометрические фигуры разных классов;
- команды пункта главного меню Show позволяют показать или стереть текущую фигуру или все фигуры, сохраняемые в списке;
- две команды пункта Scale позволяют изменить масштаб фигуры (увеличить ее или уменьшить);
- команды пункта Move позволяют перемещать текущую фигуру в четырех направлениях;
- команды пункта Color позволяют либо задать цвет фигур в диалоговом окне, либо выбрать один из предопределенных цветов;
- группа команд пункта List позволяет помещать текущую фигуру в список, перемещаться по списку и удалять из списка ту или иную фигуру;
- командные кнопки инструментальной панели соответствуют наиболее важным командам меню;
- реализована возможность перетаскивания фигур по экрану мышью.
В заключение взгляните, как выглядит форма в процессе работы с объектами:
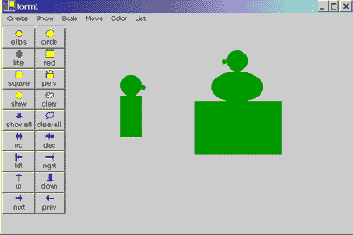
Рис. 25.1. Финальный проект. Форма в процессе работы